How to get lines of code removed from a git repository
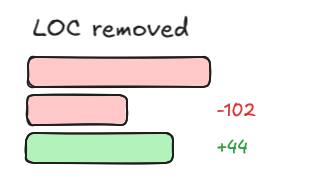
In many companies, there is an incentive to remove old code and refactor to use newer technologies or languages to make the solution smaller, easier to comprehend and maintain. If you are in a company that has goals to remove old code, it may be the case where you need to report on how many lines of code have been removed as part of your work. Here's how you might leverage Powershell to get the lines of code that you've removed from a Git repository.
# This script pulls a rough estimate of LOC (lines of code) changes for a given year
$ExecutingDirectory = (Get-Location).Path
$Year = (Get-Date).Year
$BeginningOfYear = "$($Year)-01-01"
$Commits = git --no-pager log --since="$BeginningOfYear" --oneline
$Lines = ($Commits | Measure-Object -Line).Lines
$Result = git diff --shortstat "HEAD~$Lines" HEAD 2>&1 # pipe any errors to the success stream so we can search the output below
# For a more accurate count, you may need to filter your commits to specific
# folders (ie. the source folder) of your repository. If so, you may want a
# line something similar to what is below. There are two places where you will
# need to uncomment this line
# $Result = git diff --shortstat --relative=src/ "HEAD~$Lines" HEAD 2>&1
# If we have a new repository that was created during this year, our git log
# command will return an error. The solution is to reduce the lines by 1 and
# re-run the git diff command
if ($Result | Select-String -Pattern ".*path not in the working tree.*") {
$Lines = $Lines - 1;
$Result = git diff --shortstat "HEAD~$Lines" HEAD 2>&1
# $Result = git diff --shortstat --relative=src/ "HEAD~$Lines" HEAD 2>&1
}
"Repo at '$($ExecutingDirectory)' stats for $($Year):$($Result)"
Using Powershell to get the lines of code removed from a Git repository
The output you will get when running the Powershell code above, will look something like the below.
Repo at 'C:\Users\user\repos\projectx' stats for 2024: 48 files changed, 21074 insertions(+), 1 deletion(-)